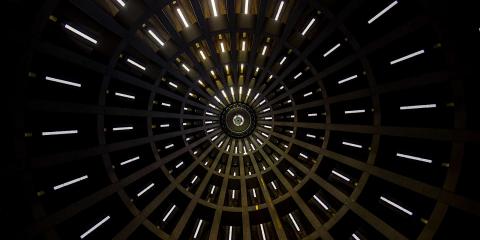
Whether you have made your start with an entry-level language like Python, Ruby, PHP, or Javascript, or a "real" language like C, or [gasp] Java, there are some compelling reasons why you should consider Go as your second language.
Many developers who have started with a common entry-level language automatically look to the most popular options for their second language; options like C++, Java, C# (ewww), Swift, or Rust. While every situation is different, in most cases, those are not the best choices.
The following are some good points why I believe you should learn Go:
Go is a Simple Language
Think simple not in the sense of limited but rather reduced complexity.
Go is primarily a structured/procedural language, without all the overbearing object oriented extra junk that encumbers many other languages. (Yes, you guessed it: although it has it's place, I'm not a fan of OO programming.)
I won't get into a big discussion about structured/procedural programming vs OO (that could go on for days!) Although you can still use easily Go in an object oriented way, and many people do, you are not forced to. There are no classes, no inheritance, no polymorphism, etc. And you are not required to write 50 lines of boilerplate code before you can even start your actual program logic.
The important point is this: Go is a simple language, but it does not sacrifice power or flexibility. There are not generally ten million ways to do the same thing (I'm looking at you, Javascript), but there are all the basics you need to write very powerful and easy to read code.
Because of this, it is easier and faster to learn and become reasonably productive with Go than with many other languages.
Go is FAST
Although for many (most?) programs, speed isn't a major or defining factor, and interpreted languages like Python, Ruby, Javascript, PHP are perfectly suitable, sometimes you do need some raw performance. Go gives you this. Performance is very close to C/C++, and in some cases orders of magnitude faster than some other languages.
Go has Lightweight Frameworks
Most frameworks for Go are built with simplicity in mind, and like Go, are easy to learn and begin using. Although there are some large and full featured web frameworks out there (Buffalo and the like), many of them are simpler, and you aren't just automatically herded into a huge framework like Laravel/Django for simple web apps. Most of the Go frameworks are abstractions on top of the built in (and superb) Go standard libraries (like net/http
), and they help you focus on your code, rather than on meeting the demands of interacting with a heavy framework.
Go Supports Powerful Concurrency
Concurrency is all the rage these days. Multiprocessing and parallelism are staples of high performance and distributed applications. Go has built in concurrency features, that are reasonably easily managed. With Go, it's sometimes only a few extra lines of code that let you turn many single threaded apps into concurrent processing power monsters. This feature makes Go highly desirable for heavy web applications and big data tasks.
But, you say, "Other languages like Rust and C++ can do concurrency too." Yes, of course they can, but not with the simplicity and ease of implementation of Go.
go func() {
//do all my concurrent stuff!
}
Go Gives YOU Control
(But not too much.)
C and C++ give you enormous amounts of control---you can do anything with them. But on the flip side you have to do everything with them. Go lets you learn to think about memory optimization, and offers you powerful ways to access objects in memory (pointers), but unlike C/C++, you are not required to deal with actual memory allocations, freeing memory up after use, garbage collection, etc. Rust (an excellent lanaguage) also gives you these abilities, but Rust is harder to learn and like, C++, is a complex language.
This ability in Go to think about how your program uses memory, and similar low-level concepts, is extremely valuable for learning how to write good code (even in other languages that don't offer the flexibility of Go).
Go is Typed
(But not too strict.)
Types are also a valuable thing to know about. They help your programs avoid some crashes and unexpected behaviors. Go makes you use them, and think about the data your program is operating on, but isn't so strict that it makes it difficult. Go has type inference, so you can be lazy sometimes and not explicitly specify a type and the compiler will do it for you, but the type is there, and will keep things "safe".
var myText string //manually set type to string
myText = "Hello!"
yourText := "Hiya!" //type inferred as string
Go Documentation is Great
In my opinion, one of the best things about Go is the official documentation: it's very good. All the standard libraries are heavily commented, and go into detail explaining how things work. The simplicity of the language itself makes good documentation easier to write and maintain.
Go has Canonical Style
Since Go is a simpler and more opinionated language than some others, and there are less ways to accomplish the same thing, it is often easier to find useful documented solutions online that fit your use case than with other languages.
For instance, look at the following question on Stack Overflow: https://stackoverflow.com/questions/3010840/loop-through-an-array-in-javascript
All this poor guy needs to do is loop over an array. Simple, right? Well, yes, but in Javascript, there are for loops, for-in, for-of, forEach, jquery.Each, map() etc. etc. The list goes on and on with 40+ answers to the guy's question. "Don't use this one for ES6", "This one is more efficient, but some people like the style of this one better...." AAAAAAAAAHHHHHH! With Golang, if you Google how to do the same thing, you'll find many answers, but they'll all be pretty much the same: use a standard for loop. It's fast; it's simple; it works.
Go is Valuable
Golang developers consistently rank in the highest paid software developer brackets. I suspect this will be the case for a long while as Go continues to take hold in the market. If you are looking to make a career in software development, Go is clearly a good choice for your future.
Go is Cross Platform
Writing in Go allows you to create programs that run on Linux, Mac, Windows, and other platforms, with zero extra effort. If you want a Windows binary, just compile your existing code using Go in Windows. If you want a Linux binary, compile it on Linux. Your same code will compile across all supported Go platforms, and produce a single binary for that platform (which is easy to distribute and needs no installation).
Go is Perfect for the Web
Web development is hot right now, and with good reason. Web apps are powering more and more facets of computing and user interfaces than ever before. Go was built with the web in mind. net/http
is part of the standard library, and isn't just a barebones web server implementation that lets you test---it's a production ready fast and powerful concurrent web server that has all the capabilities needed to deploy in pretty much any service environment.
Go is Loved
Go is one of the top 5 most loved languages. I enjoy developing in Go; I don't feel like I'm "fighting" with the language like I do when I am forced to use Javascript or some others.
func main() {
fmt.Println("Go is Awesome.")
}
So there you have it. Go is a fantastic easy-to-learn language, with some really good points, including lots of power and flexibility, without much danger or complexity or fluff, and great documentation and community. There are many language options out there, and I've seen many developers go with a default just because it is offered in a bootcamp or is very popular, and that is not always the right choice. Python remains the king of first languages, and it is a good general purpose language for many things. But hopefully the information I've shared here will give you some insight into why Go should be your choice, or at least very high on your list of choices, for a second language.
Have comments or questions? Let me know below.
Add new comment